4.2.3
Trees
Trees
Trees
Unlike other data structures in computer science, trees represent data hierarchically.


Roots and branches
Roots and branches
- Trees begin with a ‘root’ node, with one or more ‘branches’ beneath it.
- Each branch, in turn, can have one or more sub-branches connected to it.


Tree construction
Tree construction
- The rules for creating a tree are:
- Each node must only be connected to one ‘parent’ node and one or more ‘children’.
- There should be no connections directly between nodes on the same level of the hierarchy.


Tree traversal
Tree traversal
- There are three ways to navigate, or ‘traverse’ a tree data structure:
- Pre-order.
- In-order.
- Post-order.


Pre-order traversal
Pre-order traversal
- Pre-order traversal starts with the root node, then visits each of the other nodes from left to right.
- In the example shown, the nodes are visited in the following order:
- Tiger, Zebra, Lion, Gazelle, Giraffe, Rhino, Hippo, Elephant


In-order traversal
In-order traversal
- In-order traversal starts with the nodes in the left subtree, then visits the root node followed by the nodes in the right subtree.
- In this case, the nodes above are visited in the following order:
- Lion, Zebra, Gazelle, Giraffe, Tiger, Elephant, Hippo, Rhino.


Post-order traversal
Post-order traversal
- Post-order traversal starts by visiting the nodes in the left subtree from bottom to top, then the right subtree bottom to top, before finishing with a visit to the root node. - The nodes in the example above are therefore visited in the following order:
- Lion, Gazelle, Giraffe, Zebra, Elephant, Hippo, Rhino, Tiger.
Binary Search Trees
Binary Search Trees
A binary search tree is a tree data structure used for efficient searching of data.


Binary search trees
Binary search trees
- Binary search trees share the same properties as trees, with the exception that each node can have no more than two ‘child’ nodes.
- Binary search trees are very quick to search.


Storing data
Storing data
- After storing the first piece of data in the root node:
- The second entry is stored in a child node to the left of the root if it has a lesser value.
- The second entry is stored in a child node to the right if it has a greater value.


Example
Example
- The procedure for storing ‘Tiger’, ‘Zebra’, ‘Lion’, ‘Gazelle’ and ‘Rhino’ in the order given is as follows:
- 'Tiger’ takes the root node.
- ‘Zebra’ is greater than ‘Tiger’ alphabetically, so is stored as a child node to the right of the root.


Step 2
Step 2
- ‘Lion’ comes before ‘Tiger’ and so is stored as a child node to the left of the root.


Step 3
Step 3
- ‘Gazelle’ comes before ‘Tiger’ and before ‘Lion’, so is stored as a left child to the ‘Lion’ node.
- ‘Rhino’ also comes before ‘Tiger’, but after ‘Lion’ so is stored on the right of this node.
1Components of a Computer
1.1Structure & Function of the Processor
1.2Types of Processors
2Software & Software Development
2.1Systems Software
2.2Applications Generation
2.3Software Development
3Exchanging Data
3.1Compression, Encryption & Hashing
3.3Networks
4Data Types, Data Structures & Algorithms
4.1Data Types
5Legal, Moral, Cultural & Ethical Issues
5.1Computing Related Legislation
6Elements of Computational Thinking
6.1Thinking Abstractly
6.2Thinking Procedurally
6.3Thinking Logically
7Problem Solving & Programming
7.1Programming Techniques
7.2Programming Construction
Jump to other topics
1Components of a Computer
1.1Structure & Function of the Processor
1.2Types of Processors
2Software & Software Development
2.1Systems Software
2.2Applications Generation
2.3Software Development
3Exchanging Data
3.1Compression, Encryption & Hashing
3.3Networks
4Data Types, Data Structures & Algorithms
4.1Data Types
5Legal, Moral, Cultural & Ethical Issues
5.1Computing Related Legislation
6Elements of Computational Thinking
6.1Thinking Abstractly
6.2Thinking Procedurally
6.3Thinking Logically
7Problem Solving & Programming
7.1Programming Techniques
7.2Programming Construction
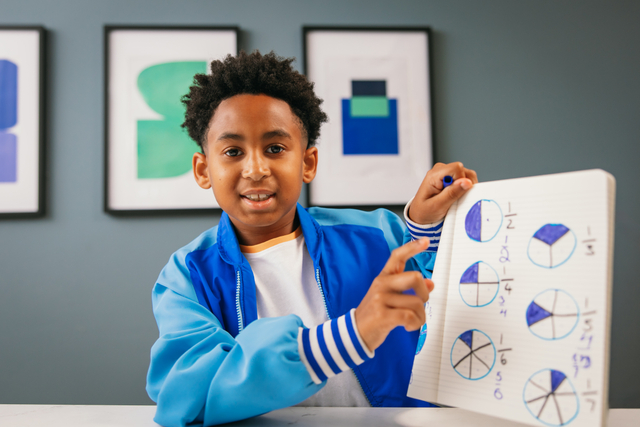
Unlock your full potential with GoStudent tutoring
Affordable 1:1 tutoring from the comfort of your home
Tutors are matched to your specific learning needs
30+ school subjects covered